ref属性
ref
被用来给元素
或子组件
注册引用信息(id的替代者
)
- 应用在
html
标签上获取的是真实DOM元素
,应用在组件标签上获取的是组件实例对象vc
- 使用方式
- 打标识:
<h1 ref="xxx"></h1>
或<School ref="xxx"></School>
- 获取:
this.$refs.xxx
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <template> <div> <h1 v-text="msg" ref="title"></h1> <button ref="btn" @click="showDOM">点我输出上方的DOM元素</button> <School ref="sch" /> </div> </template>
<script> import School from './components/School'
export default { name: 'App', components: { School }, data() { return { msg: '欢迎学习Vue!' } }, methods: { showDOM() { console.log(this.$refs.title) console.log(this.$refs.btn) console.log(this.$refs.sch) } }, } </script>
|
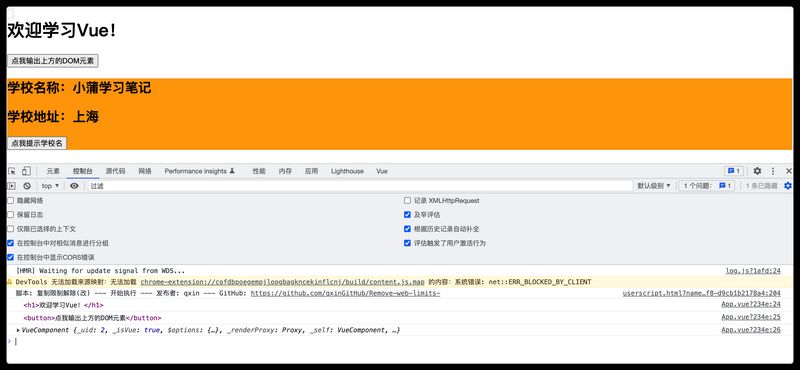
props配置项
props
让组件接收外部传过来的数据
- 传递数据
<Demo name="xxx" :age="18"/>
这里age
前加:
,通过v-bind
使得里面的18
是数字
- 接收数据
- 第一种方式(只接收)
props:['name', 'age']
- 第二种方式(限制类型)
props:{name:String, age:Number}
- 第三种方式(限制类型、限制必要性、指定默认值)
- 通过
props
传递数据给vc
后,每个vc
的数据都是独立的
1 2 3 4 5 6 7
| props: { name: { type: String, required: true, default: 'cess' } }
|
备注:props是只读的
,Vue
底层会监测你对props
的修改,如果进行了修改
,就会发出警告
,若业务需求确实需要修改,那么请复制props
的内容到data
中,然后去修改data
中的数据
src/App.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <template> <div> <Student name="李四" sex="女" :age="18"/> <Student name="王五" sex="男" :age="18"/> </div> </template>
<script> import Student from './components/Student'
export default { name:'App', components:{ Student } } </script>
|
src/components/Student.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
| <template> <div> <h1>{{ msg }}</h1> <h2>学生姓名:{{ name }}</h2> <h2>学生性别:{{ sex }}</h2> <h2>学生年龄:{{ myAge + 1 }}</h2> <button @click="updateAge">尝试修改收到的年龄</button> </div> </template>
<script> export default { name: "Student", data() { console.log(this); return { msg: "我是一个学生", myAge: this.age, }; }, methods: { updateAge() { this.myAge++; }, },
props: { name: { type: String, required: true, }, age: { type: Number, default: 99, }, sex: { type: String, required: true, }, }, }; </script>
|
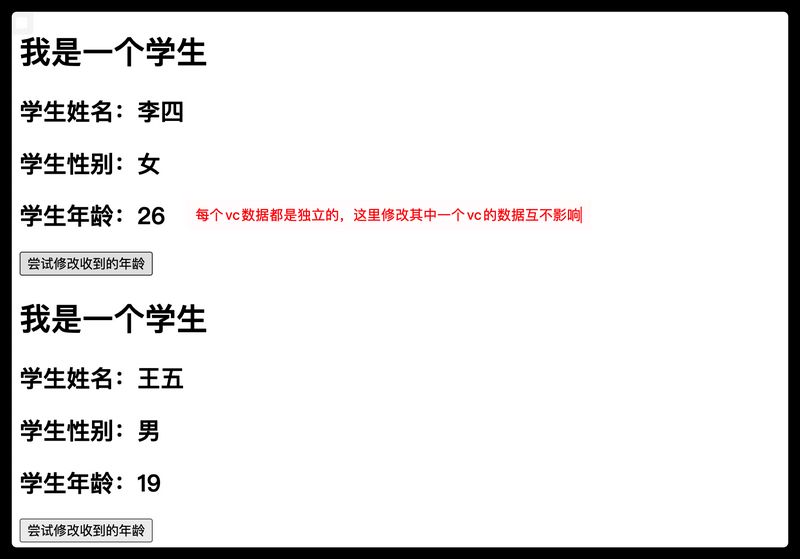
mixin 混入
功能:可以把多个组件共用的配置提取成一个混入对象
使用方式
定义混入
1 2 3 4 5
| const mixin = { data() {....}, methods: {....} .... }
|
使用混入
- 全局混入
Vue.mixin(xxx)
- 局部混入
mixins:['xxx']
备注
1、组件和混入对象含有同名选项时,这些选项将以恰当的方式进行“合并”,在发生冲突时以组件优先
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| var mixin = { data: function () { return { message: 'hello', foo: 'abc' } } }
new Vue({ mixins: [mixin], data () { return { message: 'goodbye', bar: 'def' } }, created () { console.log(this.$data) } })
|
2、同名生命周期钩子将合并为一个数组,因此都将被调用。另外,混入对象的钩子将在组件自身钩子之前调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| var mixin = { created () { console.log('混入对象的钩子被调用') } }
new Vue({ mixins: [mixin], created () { console.log('组件钩子被调用') } })
|
src/mixin.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| export const hunhe = { methods: { showName(){ alert(this.name) } }, mounted() { console.log('你好啊!') }, }
export const hunhe2 = { data() { return { x:100, y:200 } }, }
|
src/components/School.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <template> <div> <h2 @click="showName">学校名称:{{name}}</h2> <h2>学校地址:{{address}}</h2> </div> </template>
<script> import {hunhe,hunhe2} from '../mixin'
export default { name:'School', data() { return { name:'小蒲', address:'上海', x:666 } }, mixins:[hunhe,hunhe2] } </script>
|
src/components/Student.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <template> <div> <h2 @click="showName">学生姓名:{{name}}</h2> <h2>学生性别:{{sex}}</h2> </div> </template>
<script> import {hunhe,hunhe2} from '../mixin'
export default { name:'Student', data() { return { name:'张三', sex:'男' } }, mixins:[hunhe,hunhe2] } </script>
|
src/App.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <template> <div> <School/> <hr> <Student/> </div> </template>
<script> import School from './components/School' import Student from './components/Student'
export default { name:'App', components:{School,Student} } </script>
|
src/main.js
1 2 3 4 5 6 7 8 9 10 11 12
| import Vue from 'vue' import App from './App.vue'
Vue.config.productionTip = false
new Vue({ el:"#app", render: h => h(App) })
|
scoped样式
作用:让样式在局部生效,防止冲突
写法:<style scoped>
Vue
中的webpack
并没有安装最新版,导致有些插件也不能默认安装最新版,如 npm i less-loader@7
,而不是最新版
src/components/School.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <template> <div class="demo"> <h2 class="title">学校名称:{{ name }}</h2> <h2>学校地址:{{ address }}</h2> </div> </template>
<script> export default { name:'School', data() { return { name:'小蒲学习笔记', address:'上海', } } } </script>
<style scoped> .demo{ background-color: skyblue; } </style>
|
src/components/Student.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| <template> <div class="demo"> <h2 class="title">学生姓名:{{ name }}</h2> <h2 class="atguigu">学生性别:{{ sex }}</h2> </div> </template>
<script> export default { name: 'Student', data() { return { name: '张三', sex: '男' } } } </script>
<style lang="less" scoped> .demo { background-color: pink; .atguigu { font-size: 40px; } } </style>
|
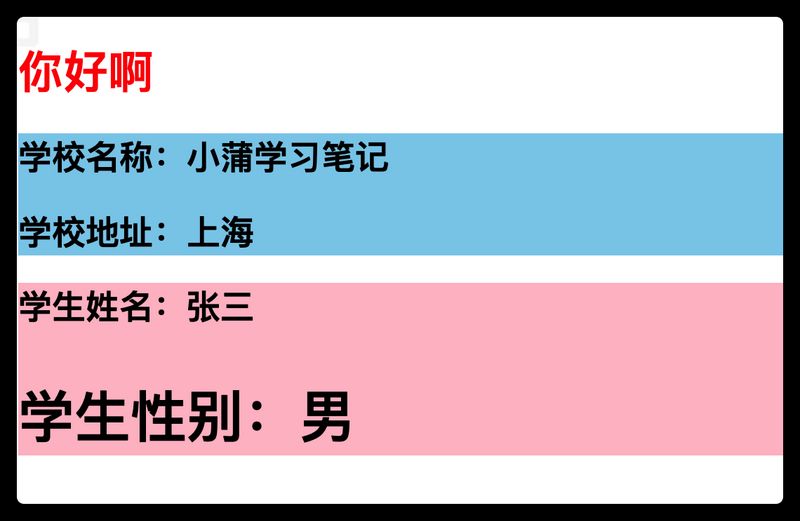