模块与组件、模块化与组件化
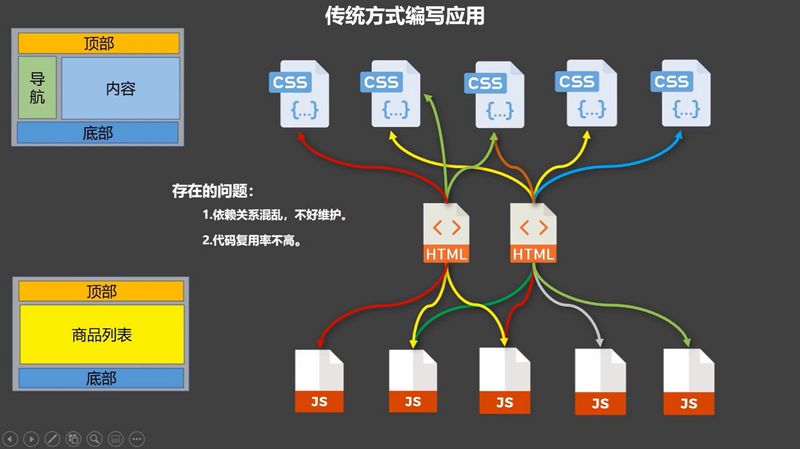
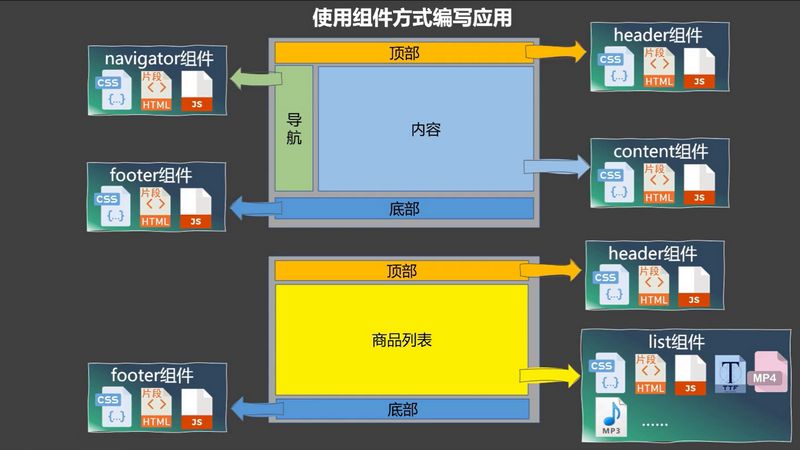
模块
- 理解:向外提供特定功能的 js 程序,一般就是一个 js 文件
- 为什么:js 文件很多很复杂
- 作用:复用、简化 js 的编写,提高 js 运行效率
组件
- 定义:用来实现局部功能的代码和资源的集合(html/css/js/image…)
- 为什么:一个界面的功能很复杂
- 作用:复用编码,简化项目编码,提高运行效率
模块化
- 当应用中的 js 都以模块来编写的,那这个应用就是一个模块化的应用
组件化
- 当应用中的功能都是多组件的方式来编写的,那这个应用就是一个组件化的应用
非单文件组件
- 非单文件组件:一个文件中包含有 n 个组件
- 单文件组件:一个文件中只包含有 1 个组件
基本使用
Vue中使用组件的三大步骤
- 定义组件
- 使用
Vue.extend(options)
创建,其中options
和new Vue(options)
时传入的options
几乎一样,但也有点区别
el
不要写,因为最终所有的组件都要经过一个vm
的管理,由vm中的el
才决定服务哪个容器
data
必须写成函数,避免组件被复用时,数据存在引用关系
- 注册组件
- 局部注册:
new Vue()
的时候options
传入components
选项
- 全局注册:
Vue.component('组件名',组件)
- 使用组件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96
| <title>基本使用</title> <script type="text/javascript" src="../js/vue.js"></script>
<div id="root"> <h2>{{msg}}</h2> <hr> <school></school> <hr> <student></student> <hr> <hello></hello> <hr> </div>
<div id="root2"> <hello></hello> </div>
<script type="text/javascript"> Vue.config.productionTip = false
const school = Vue.extend({ template: ` <div class="demo"> <h3>学校名称:{{schoolName}}</h3> <h3>学校地址:{{address}}</h3> <button @click="showName">点我提示学校名</button> </div> `, data() { return { schoolName: '蒲天辉学习笔记', address: '上海' } }, methods: { showName() { alert(this.schoolName) } }, })
const student = Vue.extend({ template: ` <div> <h3>学生姓名:{{studentName}}</h3> <h3>学生年龄:{{age}}</h3> </div> `, data() { return { studentName: '张三', age: 18 } } })
const hello = Vue.extend({ template: ` <div> <h3>你好啊!{{name}}</h3> </div> `, data() { return { name: '小蒲' } } })
Vue.component('hello', hello)
new Vue({ el: '#root', data: { msg: '你好啊!' }, components: { school, student } })
new Vue({ el: '#root2', }) </script>
|
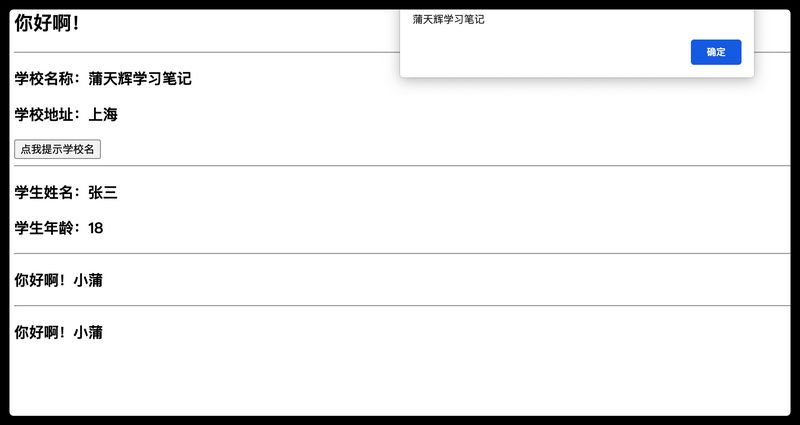
组件注意事项
关于组件名
- 一个单词组成
- 第一种写法(
首字母小写
):school
- 第二种写法(
首字母大写
):School
- 多个单词组成
- 第一种写法(
kebab-case 命名
):my-school
- 第二种写法(
CamelCase 命名
):MySchool
(需要Vue
脚手架支持)
- 备注
- 组件名尽可能回避
HTML
中已有的元素名称,例如:h2
、H2
都不行
- 可以使用
name
配置项指定组件在开发者工具中呈现的名字
关于组件标签
- 第一种写法:
<school></school>
- 第二种写法:
<school/>
(需要Vue
脚手架支持)
- 备注:不使用脚手架时,
<school/>
会导致后续组件不能渲染
一个简写方式:const school = Vue.extend(options)
可简写为const school = options
,因为父组件components
引入的时候会自动创建
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| <title>几个注意点</title> <script type="text/javascript" src="../js/vue.js"></script>
<div id="root"> <h2>{{msg}}</h2> <school></school> </div>
<script type="text/javascript"> Vue.config.productionTip = false
const school = Vue.extend({ name: 'XiaopuSchool', template: ` <div> <h3>学校名称:{{name}}</h3> <h3>学校地址:{{address}}</h3> </div> `, data() { return { name: 'XXX大学', address: '上海' } } })
new Vue({ el: '#root', data: { msg: '欢迎学习Vue!' }, components: { school } }) </script>
|
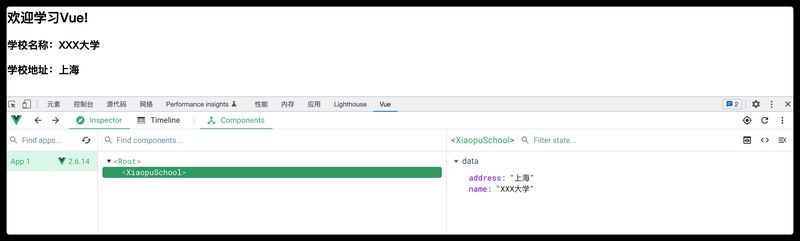
组件的嵌套
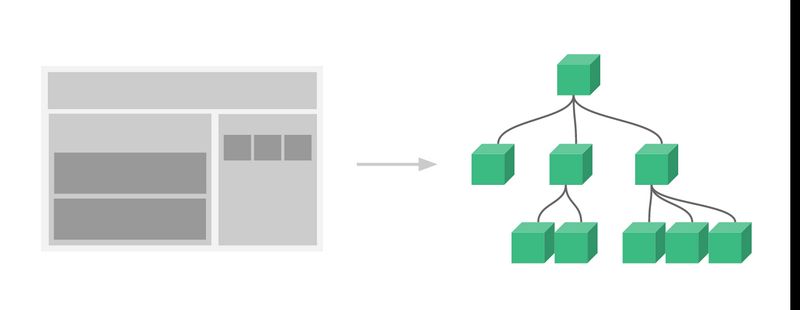
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81
| <title>组件的嵌套</title> <script type="text/javascript" src="../js/vue.js"></script>
<div id="root"></div>
<script type="text/javascript"> Vue.config.productionTip = false
//定义student组件 const student = Vue.extend({ name: 'student', template: ` <div> <h4>学生姓名:{{name}}</h4> <h4>学生年龄:{{age}}</h4> </div> `, data() { return { name: '小蒲', age: 18 } } })
//定义school组件 const school = Vue.extend({ name: 'school', template: ` <div> <h3>学校名称:{{name}}</h3> <h3>学校地址:{{address}}</h3> <student></student> </div> `, data() { return { name: '学校笔记', address: '上海' } }, //注册组件(局部) components: { student } })
//定义hello组件 const hello = Vue.extend({ template: `<h3>{{msg}}</h3>`, data() { return { msg: '欢迎来到小蒲学习笔记学习!' } } })
//定义app组件 const app = Vue.extend({ template: ` <div> <hello></hello> <school></school> </div> `, components: { school, hello } })
//创建vm new Vue({ el: '#root', template: '<app></app>', //注册组件(局部) components: { app } }) </script>
|
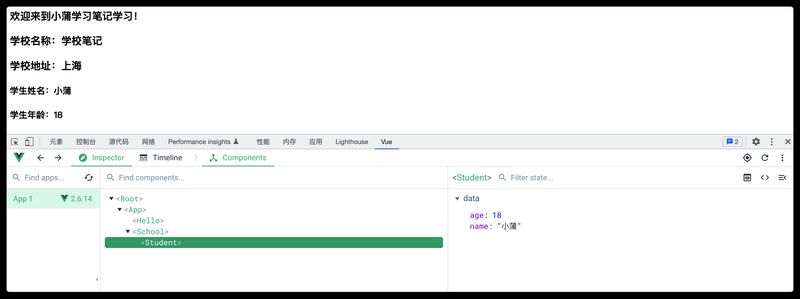
VueComponent
关于 VueComponent
school
组件本质是一个名为VueComponent的构造函数
,且不是程序员定义的,而是 Vue.extend()
生成的
- 我们只需要写
<school/>
或 <school></school>
,Vue
解析时会帮我们创建school
组件的实例对象,即Vue
帮我们执行的new VueComponent(options)
- 每次调用
Vue.extend
,返回的都是一个全新的VueComponent
,即不同组件是不同的对象
- 关于
this
指向
- 组件配置中
data
函数、methods
中的函数、watch
中的函数、computed
中的函数 它们的 this
均是 VueComponent
实例对象
new Vue(options)
配置中:data
函数、methods
中的函数、watch
中的函数、computed
中的函数 它们的 this
均是 Vue实例对象
VueComponent
的实例对象,以后简称vc
(组件实例对象
)Vue的实例对象
,以后简称vm
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| <title>VueComponent</title> <script type="text/javascript" src="../js/vue.js"></script>
<div id="root"> <school></school> <hello></hello> </div>
<script type="text/javascript"> Vue.config.productionTip = false
const school = Vue.extend({ name: 'school', template: ` <div> <h2>学校名称:{{name}}</h2> <h2>学校地址:{{address}}</h2> <button @click="showName">点我提示学校名</button> </div> `, data() {return {name: '小蒲学习笔记',address: '上海'}}, methods: {showName() {console.log('showName', this)}}, })
const test = Vue.extend({ template: `<span>xiaopu</span>` })
const hello = Vue.extend({ template: ` <div> <h2>{{msg}}</h2> <test></test> </div> `, data() {return {msg: '你好啊!'}}, components: { test } })
const vm = new Vue({ el: '#root', components: { school, hello } }) </script>
|
一个重要的内置关系
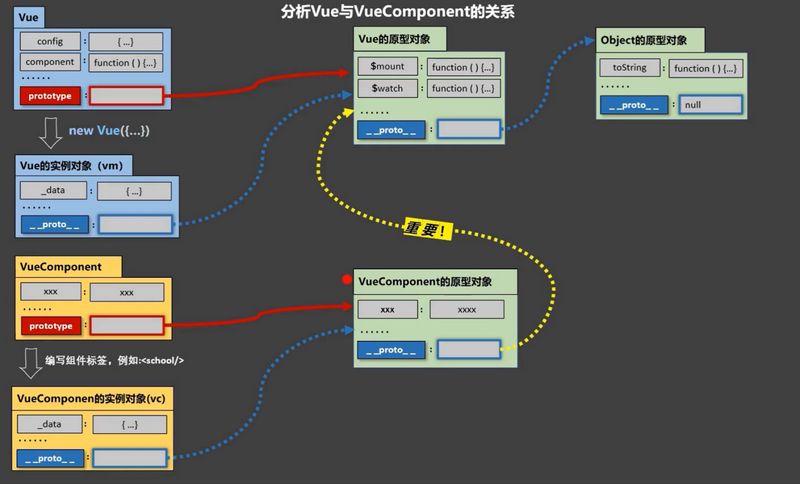
- 一个重要的内置关系:
VueComponent.prototype.__proto__ === Vue.prototype
- 为什么要有这个关系:让组件实例对象
vc
可以访问到 Vue原型
上的属性
、方法
单文件组件
School.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <template> <div id='Demo'> <h2>学校名称:{{name}}</h2> <h2>学校地址:{{address}}</h2> <button @click="showName">点我提示学校名</button> </div> </template>
<script> export default { name:'School', data() { return { name:'学习笔记', address:'上海' } }, methods: { showName(){ alert(this.name) } }, } </script>
<style> #Demo{ background: orange; } </style>
|
Student.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <template> <div> <h2>学生姓名:{{name}}</h2> <h2>学生年龄:{{age}}</h2> </div> </template>
<script> export default { name:'Student', data() { return { name:'xiaopu', age:20 } }, } </script>
|
App.vue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <template> <div> <School></School> <Student></Student> </div> </template>
<script> import School from './School.vue' import Student from './Student.vue'
export default { name:'App', components:{ School, Student } } </script>
|
main.js
1 2 3 4 5 6 7
| import App from './App.vue'
new Vue({ template:`<App></App>`, el:'#root', components:{App} })
|
index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>单文件组件练习</title> </head> <body> <div id="root"></div> <script src="../../js/vue.js"></script> <script src="./main.js"></script> </body> </html>
|